문제
Given a function fn, return a new function that is identical to the original function except that it ensures fn is called at most once.
- The first time the returned function is called, it should return the same result as fn.
- Every subsequent time it is called, it should return undefined.
https://leetcode.com/problems/allow-one-function-call/
예시
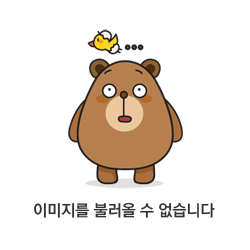
코드
type JSONValue = null | boolean | number | string | JSONValue[] | { [key: string]: JSONValue };
type OnceFn = (...args: JSONValue[]) => JSONValue | undefined
function once(fn: Function): OnceFn {
let isCalled = false;
return function (...args) {
if(isCalled) return undefined;
isCalled = true;
return fn(...args);
};
}
/**
* let fn = (a,b,c) => (a + b + c)
* let onceFn = once(fn)
*
* onceFn(1,2,3); // 6
* onceFn(2,3,6); // returns undefined without calling fn
*/
once 함수는 단 한번만 실행되어야 하는 함수이다. 처음 실행됐을 때는 인자로 들어오는 fn이 실행되어야 하고, 그 후로 인자로 fn이 들어오면 undefined가 실행되어야 한다. 따라서 isCalled라는 변수를 이용해 fn의 실행 여부를 저장하고, 한 번 실행이 되면 isCalled를 true로 변경시켰다.
728x90
'Algorithm > leetcode' 카테고리의 다른 글
[leetcode][JS] 2677. Chunk Array (0) | 2024.01.30 |
---|---|
[leetcode][JS] 2667. Create Hello World Function (0) | 2024.01.29 |
[leetcode][JS] 2665. Counter II (0) | 2024.01.25 |
[leetcode][JS] 2648. Generate Fibonacci Sequence (0) | 2024.01.25 |
[leetcode][JS] 2635. Apply Transform Over Each Element in Array (0) | 2024.01.23 |