문제
Create a class ArrayWrapper that accepts an array of integers in its constructor. This class should have two features:
- When two instances of this class are added together with the + operator, the resulting value is the sum of all the elements in both arrays.
- When the String() function is called on the instance, it will return a comma separated string surrounded by brackets. For example, [1,2,3].
https://leetcode.com/problems/array-wrapper/
예시
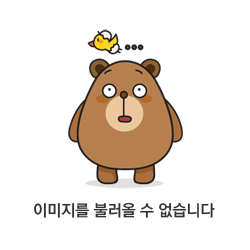
코드
/**
* @param {number[]} nums
* @return {void}
*/
var ArrayWrapper = function(nums) {
this.nums = nums;
this.sum = nums.reduce((acc, cur)=> acc + cur, 0);
};
/**
* @return {number}
*/
ArrayWrapper.prototype.valueOf = function() {
return this.sum;
}
/**
* @return {string}
*/
ArrayWrapper.prototype.toString = function() {
return "[" + String(this.nums) + "]";
}
/**
* const obj1 = new ArrayWrapper([1,2]);
* const obj2 = new ArrayWrapper([3,4]);
* obj1 + obj2; // 10
* String(obj1); // "[1,2]"
* String(obj2); // "[3,4]"
*/
ArrayWrapper라는 객체를 만들고, ArrayWrapper 끼리 덧셈을 했을 때와 String으로 표현했을 때 원하는 값이 나오도록 ArrayWrapper.prototype.valueOf()
메소드와 ArrayWrapper.prototype.toString()
메소드를 재정의했다.
ArrayWrapper 객체끼리 덧셈을 했을 때, 전체 원소의 합이 리턴되어야 한다. 따라서 각 객체를 호출했을 때의 원시 값이 해당 객체의 원소들의 합을 리턴할 수 있도록 처음 ArrayWrapper 객체를 생성할 때 sum이라는 변수에 입력 받은 초기 배열의 합을 reduce를 이용하여 계산하고 저장해놓았다. valueOf() 안에서 합을 계산할 지 고민했으나, 호출할 때마다 합을 계산하는 것이 비효율적일 것 같아서 ArrayWrapper 객체 생성 시 계산하여 저장하도록 하였다.
* Object.prototype.valueOf
객체.valueOf()
객체의 원시 값을 반환한다.
- Object는 모두 valueOf를 상속받는다.
- 객체가 원시 값을 갖고 있지 않다면, valueOf는 객체를 반환하며, 사용자 정의 객체를 만들 때는 valueOf를 재정의할 수 있다.
- 보통 원시 값을 필요로 할 때 알아서 호출된다.
function MyNumberType(n) {
this.number = n;
}
MyNumberType.prototype.valueOf = function () {
return this.number;
};
const object1 = new MyNumberType(4);
console.log(object1 + 3); // 7
* Object.prototype.toString
객체.toString()
String(객체)
객체를 나타낼 수 있는 String 값을 리턴한다.
- 객체가 텍스트 값으로 표시되거나 문자열이 예상되는 방식으로 참조될 때 자동으로 호출된다.
- Object에서 비롯된 모든 객체에 상속된다.
function Dog(name) {
this.name = name;
}
const dog1 = new Dog('Jindo');
console.log(dog1.toString()); // [object Object] -> 재정의 안 됐을 때
Dog.prototype.toString = function () {
return this.name;
}
const dog2 = new Dog('bbibbi');
console.log(dog2.toString()); // 'bbibbi'
'Algorithm > leetcode' 카테고리의 다른 글
[leetcode][JS] 2704. To Be Or Not To Be (0) | 2024.02.02 |
---|---|
[leetcode][JS] 2703. Return Length of Arguments Passed (0) | 2024.02.02 |
[leetcode][JS] 2677. Chunk Array (0) | 2024.01.30 |
[leetcode][JS] 2667. Create Hello World Function (0) | 2024.01.29 |
[leetcode][JS] 2666. Allow One Function Call (0) | 2024.01.26 |