문제
Given an integer array nums, a reducer function fn, and an initial value init, return the final result obtained by executing the fn function on each element of the array, sequentially, passing in the return value from the calculation on the preceding element.
This result is achieved through the following operations: val = fn(init, nums[0]), val = fn(val, nums[1]), val = fn(val, nums[2]), ... until every element in the array has been processed. The ultimate value of val is then returned.
If the length of the array is 0, the function should return init.
Please solve it without using the built-in Array.reduce method.
https://leetcode.com/problems/array-reduce-transformation/
예시
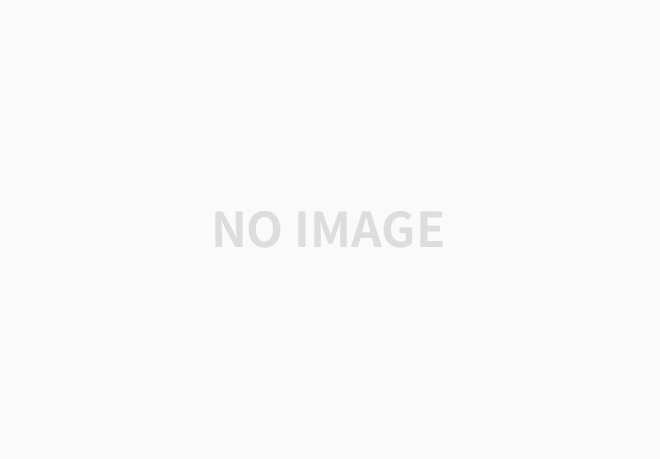
input으로 nums 배열과 reduce로 실행할 함수, 그리고 초기 값을 받는다. accum은 fn에서 리턴한 값이 들어가고, curr은 nums 배열에 있는 숫자가 들어간다.
코드
/**
* @param {number[]} nums
* @param {Function} fn
* @param {number} init
* @return {number}
*/
var reduce = function(nums, fn, init) {
if(nums.length === 0) return init;
let accum = init;
nums.forEach((num)=>{
accum = fn(accum, num);
});
return accum;
};
문제에 적혀있는 대로, nums 배열의 길이가 0이면 초기 값을 리턴한다.
fn(accum, curr)에서 accum은 앞선 실행됐던 fn의 결과 값이고, curr은 현재 nums 배열에 있는 숫자이다. for문을 돌면서 nums에서 각 숫자가 fn의 curr로 들어갈 수 있도록 하였고, fn의 결과를 accum 변수에 저장해주었다.
'Algorithm > leetcode' 카테고리의 다른 글
[leetcode][JS] 2634. Filter Elements from Array (0) | 2024.01.22 |
---|---|
[leetcode][JS] 2629. Function Composition (0) | 2024.01.22 |
[leetcode][JS] 2621. Sleep (0) | 2024.01.17 |
[leetcode][JS] 2619. Array Prototype Last (0) | 2024.01.15 |
[leetcode][JS] 2620. Counter (0) | 2023.04.20 |