문제
Given an array of functions [f1, f2, f3, ..., fn], return a new function fn that is the function composition of the array of functions.
The function composition of [f(x), g(x), h(x)] is fn(x) = f(g(h(x))).
The function composition of an empty list of functions is the identity function f(x) = x.
You may assume each function in the array accepts one integer as input and returns one integer as output.
https://leetcode.com/problems/function-composition/
예시
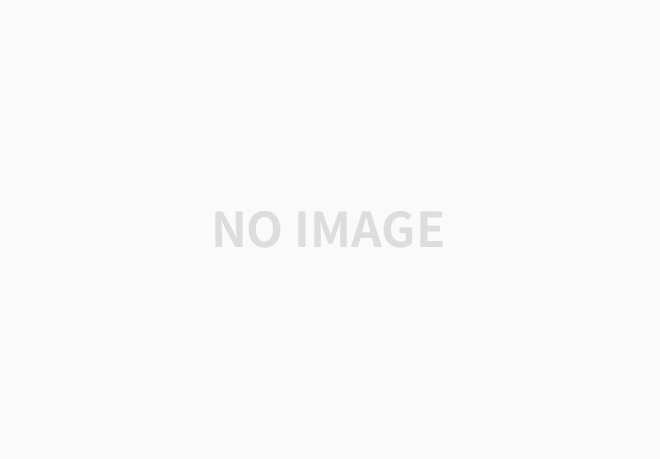
functions에 있는 각 함수들을 뒤에서부터 실행하면 된다. 주어진 x 값을 이용하여 x의 값을 업데이트한다.
코드
/**
* @param {Function[]} functions
* @return {Function}
*/
Array.prototype.myReverse = function(){
return this.map((item,idx) => this[this.length-1-idx]);
}
var compose = function(functions) {
return function(x) {
let value = x;
functions.myReverse().forEach((fn)=>{
value = fn(value);
});
return value;
}
};
/**
* const fn = compose([x => x + 1, x => 2 * x])
* fn(4) // 9
*/
함수 배열의 순서를 바꾼 뒤, 바뀐 함수 배열을 돌면서 value 값을 업데이트 했다.
Array.prototype.reverse()가 존재하지만, 직접 구현해보고 싶었다. (추가적으로, reverse()가 좀 느리지 않을까라는 생각이 들었다. 관련해서는 참고 링크를 보면 좋을 것 같다.)
참고 링크
Array.prototype.reverse 가 최선인가?
🤔 뜬금없는 의문,
medium.com
[JS] 반복문 (for, forEach 등) 속도 비교
JavaScript의 반복문으로 for loop 문, forEach 메서드, map 메서드, reduce 메서드, .each(Jquery)등정말많은종류의반복문이존재합니다.비교해볼반복문forloopforEachmapreduce.each 속도 비교에 사용할
gurtn.tistory.com
'Algorithm > leetcode' 카테고리의 다른 글
[leetcode][JS] 2635. Apply Transform Over Each Element in Array (0) | 2024.01.23 |
---|---|
[leetcode][JS] 2634. Filter Elements from Array (0) | 2024.01.22 |
[leetcode][JS] 2626. Array Reduce Transformation (0) | 2024.01.18 |
[leetcode][JS] 2621. Sleep (0) | 2024.01.17 |
[leetcode][JS] 2619. Array Prototype Last (0) | 2024.01.15 |