문제
You are given two strings word1 and word2. Merge the strings by adding letters in alternating order, starting with word1. If a string is longer than the other, append the additional letters onto the end of the merged string.
Return the merged string.
https://leetcode.com/problems/merge-strings-alternately/
예시
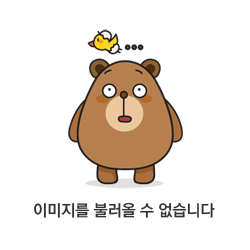
word1부터 번갈아가면서 string을 합쳐주면 된다. 이때, 더이상 번갈아 합칠 수 없으면, 긴 string의 나머지 부분은 그대로 더해진다.
코드
/**
* @param {string} word1
* @param {string} word2
* @return {string}
*/
var mergeAlternately = function(word1, word2) {
let words = "";
const minLen = Math.min(word1.length, word2.length);
// minLen 만큼은 번갈아 가면서
for(let i = 0; i < minLen; i++){
words += word1[i] + word2[i];
}
// 나머지 긴 string은 붙이기
words += word1.length > word2.length ? word1.slice(minLen) : word2.slice(minLen);
return words;
};
word1과 word2 중 짧은 길이만큼 번갈아서 더해주었다.
그 후, word1과 word2 중 더 긴 길이를 가진 string의 남은 부분을 더해주었다.
Array.prototype.slice() 를 사용하면 배열의 원하는 인덱스부터 끝까지 자를 수 있다.
728x90
'Algorithm > leetcode' 카테고리의 다른 글
[leetcode][JS] 2629. Function Composition (0) | 2024.01.22 |
---|---|
[leetcode][JS] 2626. Array Reduce Transformation (0) | 2024.01.18 |
[leetcode][JS] 2621. Sleep (0) | 2024.01.17 |
[leetcode][JS] 2619. Array Prototype Last (0) | 2024.01.15 |
[leetcode][JS] 2620. Counter (0) | 2023.04.20 |