문제
Given two arrays arr1 and arr2, return a new array joinedArray. All the objects in each of the two inputs arrays will contain an id field that has an integer value.
joinedArray is an array formed by merging arr1 and arr2 based on their id key. The length of joinedArray should be the length of unique values of id. The returned array should be sorted in ascending order based on the id key.
If a given id exists in one array but not the other, the single object with that id should be included in the result array without modification.
If two objects share an id, their properties should be merged into a single object:
- If a key only exists in one object, that single key-value pair should be included in the object.
- If a key is included in both objects, the value in the object from arr2 should override the value from arr1.
https://leetcode.com/problems/join-two-arrays-by-id/
예시
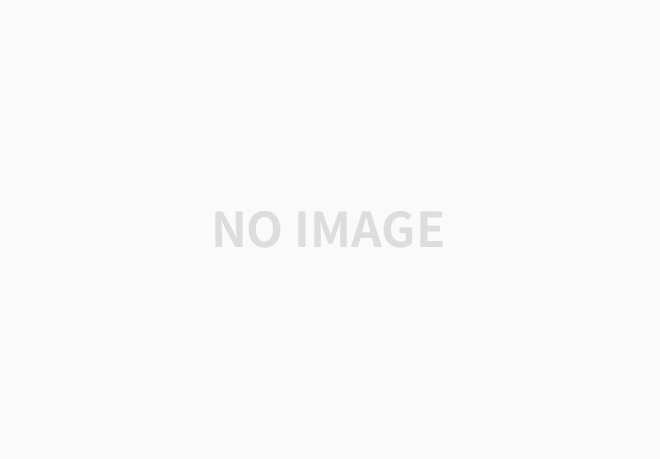
코드
/**
* @param {Array} arr1
* @param {Array} arr2
* @return {Array}
*/
var join = function(arr1, arr2) {
const hashMap = {};
for(obj of arr1.concat(arr2)){
const id = obj["id"];
if(hashMap[id]){
// id exist
hashMap[id] = {...hashMap[id], ...obj};
}else{
hashMap[id] = obj;
}
}
return Object.values(hashMap);
};
hashMap이라는 새로운 객체를 만들고, id를 key로 갖고 객체를 value로 갖도록 hashMap 형태로 만들었다. hashMap에 해당 id가 없는 경우에는 hashMap에 새로 추가했다. 이미 key가 id인 객체가 있는 경우에는 스프레드 연산자를 활용해서 기존에 있던 객체와 새로운 객체를 합쳐서 같은 key인 경우에는 뒤에 있는 객체의 value로 덮어씌울 수 있도록 했다.
문제에서 정렬을 하라고 했는데, JS는 object를 key 값 기준으로 정렬해주기 때문에 따로 정렬해주지 않았다.
그 후 Object.values()
를 활용해 values로 이루어진 배열 형태를 반환한다.
'Algorithm > leetcode' 카테고리의 다른 글
[leetcode][JS] 2721. Execute Asynchronous Functions in Parallel (0) | 2024.08.07 |
---|---|
[leetcode][JS] 2624. Snail Traversal (0) | 2024.08.01 |
[leetcode][JS] 2727. Is Object Empty (0) | 2024.02.13 |
[leetcode][JS] 2726. Calculator with Method Chaining (0) | 2024.02.11 |
[leetcode][JS] 2725. Interval Cancellation (0) | 2024.02.11 |